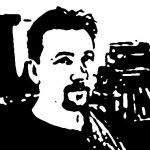
Mike Trethowan
In .Net, sliders can be frustrating in their glitchiness. As an option I have tried to create another method using buttons. I have failed in regards to this in the past, but a sleepless night turned productive and the answer came to me. Since this doesn’t require a lot of code I will keep this short.
In testing different Button1 events I realized that the button click event didn’t take place until after the mouse button was released. I decided to use this to my advantage. So, let’s start by creating a project.
Double click the form to create Private Sub Form1_Load. Next, place a label, a button and a timer on the form. Double click the button and the timer to create their subs. In the label properties set TestAlign to MiddleCenter and set AutoSize to FALSE. Drag the label so that it is over the button and the same width as the button. This will keep the text centered over the button.
Now for the code. Add an integer variable named int, (this is not a reserve word in VB.Net.) Within the sub Form1_Load add Label1.Text = “Test”
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load Label1.Text = "Test" End Sub
Next, copy the sub for Button1_Click and paste a copy below the original Button1_Click and rename it Button1_MouseDown. Change the Handler to Button1.MouseDown. Now you have two separate Button events. These events will reset int to = 0 and start and stop Timer1. Add the following code to those events.
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click ' MouseLeave Timer1.Enabled = False End Sub Private Sub Button1_MouseDown(sender As Object, e As EventArgs) Handles Button1.MouseDown int = 0 Timer1.Enabled = True End Sub
The code within Timer1 will increment int by 1 each time the timer event fires while the timer is active. Add the following code and start the program to see how well this works out.
Private Sub Timer1_Tick(sender As Object, e As EventArgs) Handles Timer1.Tick int += 1 Label1.Text = int.ToString End Sub
One can add a second button and a second timer to decrement int as one would for use in a volume up and down control as I did in my completed project.
Public Class Form1 Dim int As Integer Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load int = 0 Label1.Text = "Test" End Sub Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click ' MouseLeave Timer1.Enabled = False int += 1 Label1.Text = int.ToString End Sub Private Sub Button1_MouseDown(sender As Object, e As EventArgs) Handles Button1.MouseDown Timer1.Enabled = True End Sub Private Sub Timer1_Tick(sender As Object, e As EventArgs) Handles Timer1.Tick int += 1 Label1.Text = int.ToString End Sub Private Sub Button2_Click(sender As Object, e As EventArgs) Handles Button2.Click ' MouseLeave Timer2.Enabled = False int -= 1 Label1.Text = int.ToString End Sub Private Sub Button2_MouseDown(sender As Object, e As EventArgs) Handles Button2.MouseDown Timer2.Enabled = True End Sub Private Sub Timer2_Tick(sender As Object, e As EventArgs) Handles Timer2.Tick int -= 1 Label1.Text = int.ToString End Sub End Class
I hope that this is useful to someone out there. Happy coding.
Disclaimer: The code in this tutorial is not intended as a final product or by no means the only way this project can be coded. The presented code is for educational purposes only and no warranty is granted or implied. I/we are not responsible for any damages as a result of using this code. Use at your own risk.