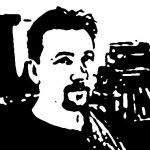
Mike Trethowan
Recently I bought a Stratux Vk-162 Remote Mount USB GPS online from Amazon. Since I have worked with various GPS modules over the years, USB and OEM boards, I decided to do a short tutorial. This is a .Net based tutorial in VB to demonstrate how to decode a NMEA sentence.
Decoding NMEA sentence can seem confusing, I have seen lots of questions on Programming User Groups, but it really isn’t. In reality, the components of the NMEA sentence are real easy to deconstruct using the .Net environment. NMEA sentence are multi part. The sentence structure always follows the form; Header, Statement(s), Checksum, and end with a “Ctrl Linefeed.” Each segment of the NMEA sentence is separated by a comma, and for many experienced programmers this is a clue to decoding. We will parse the NMEA sentence using the Split function. Another nice feature is that Windows sees these GPS modules as serial devices and assigns COM ports to them.
Start a new Visual Studio VB.Net (or C#) project. Once the projects starts, add a Timer component and set the Timer component intervals to 120. Next, add a Com Port component. Set the Com port to the address of the G-Mouse, and the baud rate to 9600.
Next, double click the form to create the first subroutine. Go back to the Designer View and double click the Timer component. Even though .Net destroys running items upon closing, you can add a Form Closing statement just the same.
Public Class Form1 Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load End Sub Private Sub Form1_Closing(sender As Object, e As EventArgs) Handles Me.Closing Try Timer1.Enabled = False SerialPort1.Close() Catch ex As Exception End Try End Sub Private Sub Timer1_Tick(sender As Object, e As EventArgs) Handles Timer1.Tick End Sub End Class
In the Code View add a new sub named Setup and add statements that will open the serial port and start the timer. In Form1_Load add the statement SetUp() to begin reading the GPS once the program loads.
Public Class Form1 Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load SetUp() End Sub Private Sub Form1_Closing(sender As Object, e As EventArgs) Handles Me.Closing Try Timer1.Enabled = False SerialPort1.Close() Catch ex As Exception End Try End Sub Private Sub Timer1_Tick(sender As Object, e As EventArgs) Handles Timer1.Tick End Sub Private Sub SetUp() Try SerialPort1.Open() SerialPort1.DiscardInBuffer() Timer1.Enabled = True Catch ex As Exception MsgBox(ex.Message) End Try End Sub Private Sub TestData(ByVal gps As String) End Sub End Class
Add a label to the form and rename it from Label1 to Label0 and change the text to Label0. There is a method to my madness. After you have done this, add ten more labels to the form and line them up in a column. Visual Studio will have named these next labels as Label1 to Label10.
When done, return to the code view and add the following code to TestData.
Private Sub TestData(ByVal gps As String) Try If gps.Length > 6 And gps.Substring(0, 6) = "$GPRMC" Then Try Dim GPS_Data As String() = gps.Split(New Char() {","c}) Label0.Text = "Message ID: " & GPS_Data(0) Label1.Text = "UTC Position: " & GPS_Data(1) Label2.Text = "Status: " & GPS_Data(2) Label3.Text = "Latitude: " & GPS_Data(3) & " " & GPS_Data(4) Label5.Text = "Longitude: " & GPS_Data(5) & " " & GPS_Data(6) Label7.Text = "Speed Over Ground: " & GPS_Data(7) Label8.Text = "Course Over Ground: " & GPS_Data(8) Label9.Text = "Date: " & GPS_Data(9) Label10.Text = "Magnetic Variation: " & GPS_Data(10) Catch ex As Exception Timer1.Enabled = False MsgBox(ex.Message) End Try End If Catch ex As Exception End Try End Sub
To save typing, just copy the above code. Once you are done, run the code. Finally we add the code that retrieves the GPS data.
Private Sub Timer1_Tick(sender As Object, e As EventArgs) Handles Timer1.Tick Try If SerialPort1.BytesToRead Then TestData(SerialPort1.ReadExisting) End If Catch ex As Exception End Try End Sub
NOTE: Here I have used SerialPort1.ReadExisting instead of SerialPort1.ReadLine. I have not looked at the raw code, but using SerialPort1.ReadLine with data from the Stratux Vk-162 doesn’t work. I just happen to have an old Pharos GPS-360 that came with Microsoft Street And Trips that I bought several years ago. With the Pharos, SerialPort1.ReadLine works but SerialPort1.ReadExisting doesn’t. If your GPS is a different model than these, you will have to play around to see which code works best.
If all works as intended your form will look similar to the image below.
I did do a GPS tutorial some time back that you may want to check out also. It is a bit cruder than this one. It also is not for a USB GPS, but for an OEM Board with an on board UART connected to a FTDI 232R USB to Serial dongle.
Tip: If you are working in Windows, you can use the Device Manager to check the assigned COM port and the baud rate of the USB GPS device that you’re are using.
Disclaimer: The code in this tutorial is not intended as a final product or by no means the only way this project can be coded. The presented code is for educational purposes only and no warranty is granted or implied. I/we are not responsible for any damages as a result of using this code. Use at your own risk.