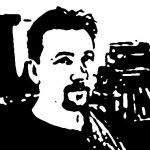
Mike Trethowan
Building on the previous post VB.NET Quick Tip – Reading A USB GPS, I will show how to use a USB to Serial cable manufactured by FTDI. In my projects, prototyping and final products I use the FTDI TTL-232R extensively. The cable is reasonably priced and comes in two varieties, a 5 volt and 3.3 volt model.
The Form will have one of each, a Label, a TextBox, a Panel a Button and a SerialPort. That’s all you will need. Like the previous Quick Tip, the serial port will be configured at startup using ManagementObjectSearcher to find and set the com port. The Serial Port will trigger an Event when there is data available and using a Cross Thread routine send the message to the Label. The message is generated using a TextBox for the inputm a Button to send the TextBox contents and a SerialPort. The ManagementObjectSearcher will require that you add a reference to your project n the Project Properties page under the References Tab.
Place all of the objects on your form and name them. Name the Label lblMessage, the TextBox txbMessage, the Button btnSend, the Panel pnlRx and make the size 14,14. Name the SerialPort spUSB.
Only one variable will be needed in this project:
#Region " Dims " Const VidPid As String = "VID_0403+PID_6001+RAYIG8GTA" ' Acquired using Device Manager #End Region
Use Windows Device Manager to get the Vendor ID and Product ID numbers. Add the routine that sets the serial port. As mentioned, the serial port is located and set using ManagementObjectSearcher. Once the searcher provides a collection of available ports loop through them and locate the device that we need the port name for. Once the device is located exit the loop. This is put in the form of a Function that returns a Boolean even though the Boolean is not used. In a more refined project the Function can be tested in an IF statement and if there are no ports found, i.e. findDevice() returns false a message can be generated for the user to take some action.
#Region " Serial Routines " Public Function FindDevice() As Boolean Dim answer As Boolean = False Dim searcher As New ManagementObjectSearcher("root\WMI", "SELECT * FROM MSSerial_PortName") For Each queryObj As ManagementObject In searcher.Get() Dim foundDevice As Integer = queryObj("InstanceName").IndexOf(VidPid) If foundDevice > -1 Then lblMessage.Text = "GPS Found At " & queryObj("PortName") answer = True spUSB.PortName = queryObj("PortName") System.Threading.Thread.Sleep(100) spUSB.Open() Exit For End If Next Return answer End Function #End Region
GetMessage() and SendMessage() are simple routines, not much different from the GetMessage() from the previous post. Place bothe of these in the “Serial Routines” region with FindDevice()
Private Sub GetMessage(sender As Object, e As SerialDataReceivedEventArgs) Try If spUSB.IsOpen And spUSB.BytesToRead Then DisplayMessage(lblMessage, spUSB.ReadLine) pnlRx.BackColor = Color.Green Else pnlRx.BackColor = Color.Red spUSB.Open() End If Catch ex As Exception DisplayMessage(lblMessage, "Error In GetMessage() " & ex.Message) End Try End Sub Private Sub SendMessage(ByVal txMessage As String) ' Translate the passed message into ASCII and store it as a byte array. Try If spUSB.IsOpen Then spUSB.WriteLine(txMessage) Catch ex As Exception lblMessage.Text = "Error In SendMessage() " & ex.Message End Try End Sub
When the program runs we want the StartUp() routine to be called from the code for the Form Load Event, so add this code and call it when the program loads. Remember, double clicking the main form will automatically generate the code for the Form Load Event.
Private Sub StartUp() lblMessage.Text = "Status: Ready" bgwGetMessage.WorkerSupportsCancellation = True FindDevice() AddHandler spUSB.DataReceived, AddressOf GetMessage End Sub
And finally, add the code in the Button Click Event.
#Region " Button Events " Private Sub btnSend_Click(sender As Object, e As EventArgs) Handles btnSend.Click If txbMessage.Text <> "" Then SendMessage(txbMessage.Text) End Sub #End Region
Now simply jump the Orange and Yellow pins on the TTL-232R, plug the cable into the USB Port and run your program. Type a message in txbMessage, press the Send button and you should see it Echoed in lblMessage. Here are the screen shots of my program at startup, after I typed the message into the text box and after sending the message. Messages can be sent in any form. Projects that can be designed from this include Microcontroller Interfacing to the PC, Weather Station, Robotics and Home Automation.
Happy Coding.
Disclaimer: The code in this tutorial is not intended as a final product or by no means the only way this project can be coded. The presented code is for educational purposes only and no warranty is granted or implied. I/we are not responsible for any damages as a result of using this code. Use at your own risk.